教程視頻:
- 結文結構:
- 1.MongoDB常用的查詢條件介紹
- 2.使用find指令範例(基礎)
- 3.條件操作符的使用
在介紹『條件操作符』之前先要了解一下 find 指令,我們來看一下 find 指令的結構。
db.collection.find(
{條件},
{鍵指定}
)
參數說明
條件 :條件操作符物件
鍵指定:想要顯示的欄位
在使用 find 前我們需要準備一些資料,以利學習,我們要下載一下範本的資料表,然後使用mongoimport指令將資料匯入我們的資料庫中。
操作步驗如下:
1.下載 JSON file (http://media.mongodb.org/zips.json)
2. 匯入資料
mongoimport.exe --db mydb --file ../zips.json
connected to: 127.0.0.1
no collection specified!
using filename 'zips' as collection.
2015-01-12T09:30:55.804+0800 check 9 29353
2015-01-12T09:30:55.811+0800 imported 29353 objects
3.當出現imported 29353 時則表示資料已成功匯入了,我們再使用
mongo指令連接到你的server中查到一下。
3.1 使用use mydb 切換資料庫
3.2 使用 db.zips.find().count() 是否有輸出有 29353 筆資料
*使用find指令範例(基礎)
1.查詢全部的資料
db.zips.find()
或是
db.zips.find({ })
查詢結果如下圖
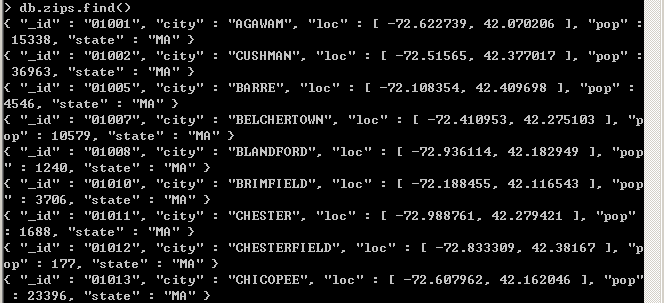
注意:mongo在查詢資料時侯是有限制顯示筆數。有像是20筆,
如你要看下一頁的資料請鍵入 it 後按下 Enter 鍵即可,查看下一頁。
2.限制顯示的欄位
這一個操作就像是SQL中的顯示數位
SELECT CITY FROM ZIPS
在find的指令的第2個引數就是來完成相同的操作,但有一點必須注意就
是 _id 是預設顯示的,如果你不想看見的話,把他設定成 0 。 如下操作
db.zips.find({},{_id:0 , city:1})
* 0 表示不要顯示此欄位
1 表示要顯示此欄位
查詢結果如下圖
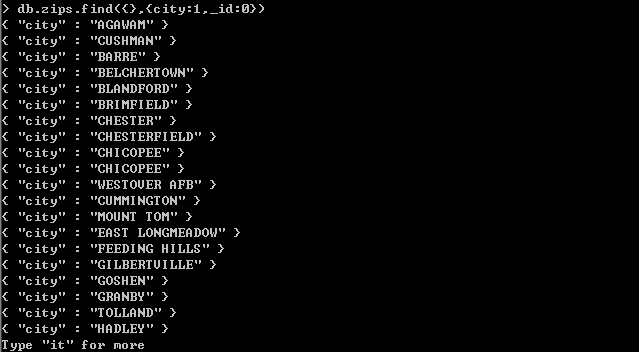
3.限制顯示的欄位 + 排序
使用sort指令直接連綴在find指令之後,就像是JQuery的語法。
sort的格式為
( {欄位:排序方式} )
*排序方式 等於 『 1 』時表示正向排序就是SQL中的ASC
*排序方式等於 『- 1』時表示反向排序就是SQL中的DESC
db.zips.find({},{city:1,_id:0}).sort({city:1})
查詢結果如下圖
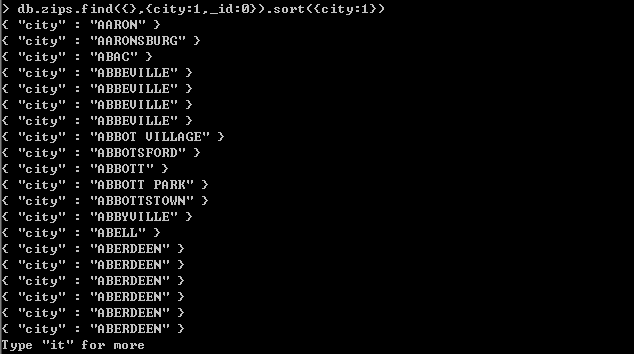
現在大家都應該了解基本的find指令的運用了吧,接下來就是條件操作符的使用,以下內文擷於http://docs.mongodb.org/manual/reference/operator/query/,在這篇文章的內容說真的要詳細說明的話要花費不少的時間,所以我只針對find指令會用到的條件操作符做說明( Comparison , Logical , Element , Evluation),另外黃底部份是我額外的說明或程式碼範例。
Comparison
For comparison of different BSON type values, see the specified BSON comparison order.
Name | Description |
---|---|
$gt | Matches values that are greater than the value specified in the query. 大於 ( > ) 使用範例:
將返回 4 筆資料 |
$gte | Matches values that are greater than or equal to the value specified in the query. 大於等於 ( >= )使用範例:
將返回 4 筆資料 |
$in | Matches any of the values that exist in an array specified in the query. 包含 使用範例:
將返回 40 筆資料 |
$lt | Matches values that are less than the value specified in the query. 小於 ( < ) 使用範例: db.zips.find( { pop: { $lt:100 } }).count() 將返回 701 筆資料 |
$lte | Matches values that are less than or equal to the value specified in the query. 小於等於 ( <= ) 使用範例:
將返回 706 筆資料 |
$ne | Matches all values that are not equal to the value specified in the query. 不等於 ( != ) 使用範例:
將返回 29313 筆資料 |
$nin | Matches values that do not exist in an array specified to the query. 不包含使用範例:
將返回 29313 筆資料 |
Element
Logical
Name | Description |
---|---|
$and | Joins query clauses with a logical AND returns all documents that match the conditions of both clauses. 並且 ( && ) db.zips.find({ $and:[ {city:"AGAWAM"}, {pop:15338} ] }) |
$nor | Joins query clauses with a logical NOR returns all documents that fail to match both clauses. 或取反 ! ( or ) ,也就是找不符合條件的資料
將返回 87 筆資料 |
$not | Inverts the effect of a query expression and returns documents that do not match the query expression. 返選操作 *取得city 不包含 NEW 字眼的所有城市 將返回 28879 筆資料 |
$or | Joins query clauses with a logical OR returns all documents that match the conditions of either clause. 或 ( or )
|
Evaluation
Name | Description |
---|---|
$mod | Performs a modulo operation on the value of a field and selects documents with a specified result. |
$regex | Selects documents where values match a specified regular expression. 使用正則式查詢
|
$text | Performs text search. |
$where | Matches documents that satisfy a JavaScript expression. 進階的查詢功能,一但使用到這一個功能查詢的速度可以會下降,但他的功能算是更強大的 db.zips.find({ $where: function() { if(this.state=='MA' && this.city.indexOf('EAST') >= 0 ){ return true; } if(this.state=='WA' && this.city.indexOf('EAST') >= 0 ){ return true; } return false; } })返回的資料筆數為 18 筆 。 |
Name | Description |
---|---|
$exists | Matches documents that have the specified field. 欄位存在檢查操作 我們先執行一個更新操作
我們必須先更新 _id 為 01001 這一筆資料,插入一個新的欄位為 test;接下來我們可以用測式exists指令了,我們要找有test欄位的 文檔就可以透過下列指令完成。
返回資料為: { "_id" : "01001", "city" : "AGAWAM", "loc" : [ -72.622739, 42.070206 ], "pop" : 15338, "state" : "MA", "test" : "test" } |
$type | Selects documents if a field is of the specified type. 請參考 http://docs.mongodb.org/manual/reference/operator/query/type/ ,因為這一個操作比較少使用,我就不多說了。 |
最後出幾個綀習題
1.在zips中查出不重覆的city名稱,並且使用city作排序由小到大
> db.zips.distinct("city").sort({city:1})
2.在zips中查出status 為 WA 或 AL 的所有城市名稱?
> db.zips.find({ state :{$in:['WA','AL']} }).count()
3.在zips中查出_id值大於等於10000 並且 小於等於 100100 的city有那一些?
> db.zips.find({ $and:[{ _id : { $gt:'10000' }},{_id:{$lt:'100100'}}] })
4.使用正則式找到 city 以EAST 開頭 ,並且結尾字母為N的城市?
4.使用正則式找到 city 以EAST 開頭 ,並且結尾字母為N的城市?
> db.zips.find({city:/^EAST.+N$/i}).count()
PS:不熟悉正則表達式的人可以 參考一下這篇文章
http://openhome.cc/Gossip/JavaGossip-V1/RegularExpression.htm
PS:不熟悉正則表達式的人可以 參考一下這篇文章
http://openhome.cc/Gossip/JavaGossip-V1/RegularExpression.htm
下節教程內容為 MongoDB的條件操作符(2)
有個小錯誤,$lte的範例部分寫錯了
回覆刪除